
Using Traefik Reverse Proxy For Securing Microservices On Azure Service Fabric
Service Fabric is a Microservices platform by Microsoft, similar to Docker Swarm/Kubernetes. It provides great features out of the box and helps orchestrate and manage your microservices.
When you deploy your web applications to Service Fabric, it's a good idea to have them exposed through a reverse-proxy instead of exposing them directly to the outside world. While Microsoft does provide a reverse-proxy out of the box, it severely lacks in features and functionality.
It also exposes all your microservices running in the cluster directly to the outside world. There is no way to restrict access to certain microservices and enable access to other ones (unless you do that in the code). Its all or nothing. This has always bugged me because I shouldn't have to write custom code if I don't want to expose my services.
While there are other options like using API Managment to expose the service endpoints securely, it might be an over kill if you don't want to use many of the API management features. Its expensive and adds lot of overhead in managing the endpoints. Besides, API Management doesn't help in exposing websites, it's only for the APIs.
Thankfully, Traefik reverse proxy is an awesome tool created by extremely talented folks at Containo.us that can help us in safely exposing our services. Besides acting as a reverse proxy, it also acts as a load balancer, circuit breaker too...
It supports automatic SSL creation using Let's Encrypt, provides great metrics on how your services are being used, along with providing a clean web interface to visualizse everything. It's open-source and completely free! In short, there isn't a reason to not use such an amazing tool.
It supports quite a few backends like Docker, Kubernetes, Mesos, Consul etc., along with Service Fabric.

How does Traefik work with Service Fabric
Traefik is distributed as an .exe file that will be deployed to Service Fabric Cluster as a guest executable. The process is the same as deploying any other Microservice to the cluster.
The difference is, Traefik has a built-in Service Fabric Provider that will query the Service Fabric Management APIs to discover what services are running in the cluster (known as backends). The provider then maps the routing rules (known as frontends) to these service instances.
Traffic to the services flows through the entry points, which are then matched and mapped through the frontends. Each request is then load balanced across various service instances in the backend. The rules for load balancing can be configured on the Traefik configuration file.
It's possible to use Traefik as a very efficient HTTP load balancer to distribute traffic to several microservices to improve performance, stability and reliability of web apps/apis using it's very powerful configuration rules.
Instructions on how to use Traefic on Service Fabric are provided here:
1. Clone this repository to your local machine.
2. Download the Træfik binary into the solution by running the following PowerShell script.
3. Open the Traefik.sln file in Visual Studio
4. Træfik must authenticate to the Service Fabric management API. Currently, you can only do this using a PEM formatted client certificate. If you only have a .pfx certificate you will need to convert it using the following commands:
• Extract the private key from the .pfx file
Træfik only requires read-only access to the Service Fabric API and thus you should use a Read-Only certificate.
5. Copy your generated certificate files to the Code\certs folder Træfik expects to find them in.
6. If you wish to track the new certificate files in Visual Studio, you'll need to add them to your solution by right clicking on the $REPO_ROOT\Traefik\ApplicationPackageRoot\TreafikPkg\Code\certs folder and selecting Add Existing Item, navigate to the certificates on local disk and select Add.
7. Open ($REPO_ROOT\Traefik\Traefik\ApplicationPackageRoot\TraefikPkg\Code\traefik.toml) in a text editor. If you're using a secure cluster, ensure the TLS configuration section is uncommented and make sure the provided certificate paths are correct. Additionally, change the clustermanagementurl to use the prefix (https://).
The clustermanagementurl setting is relative to where Træfik is running. If Træfik is running inside the cluster on every node, the clustermanagementurl should be left as http[s]://localhost:19080, if however, Træfik is running externally to the cluster, an accessible endpoint should be provided. If you are testing Traefik against an unsecure cluster, like your local onebox cluster, use http://localhost:19080
8. Optional You can choose to enable a watchdog service which will report stats and check Traefik is routing by sending synthetic requests and recording the results. The results of these checks are sent to Application Insights.
9. You can now simply publish Træfik from Visual Studio like any other Service Fabric application. Right click on the project, select Publish and follow the publication wizard.
I've created two microservices that are just deployed to the local Service Fabric Cluster. The services are still being deployed to varioud nodes in the cluster.

• To be able to ingress external requests via Traefik, you'll need to open up and map the relevant ports on your public load balancer. For clusters on Azure, this will be your Azure Load Balancer. The default ports are: tcp/80 (proxy) and tcp/8080 (API) but these can be configured in $REPO_ROOT\Traefik\ApplicationPackageRoot\TraefikPkg\Code\traefik.toml and in $REPO_ROOT\Traefik\Traefik\ApplicationPackageRoot\TraefikPkg\ServiceManifest.xml.
11. Once the load balancer has been configured to route traffic on the required ports, you should be able to visit the Træfik dashboard at http[s]://[clusterfqdn]:8080 if you have it enabled.
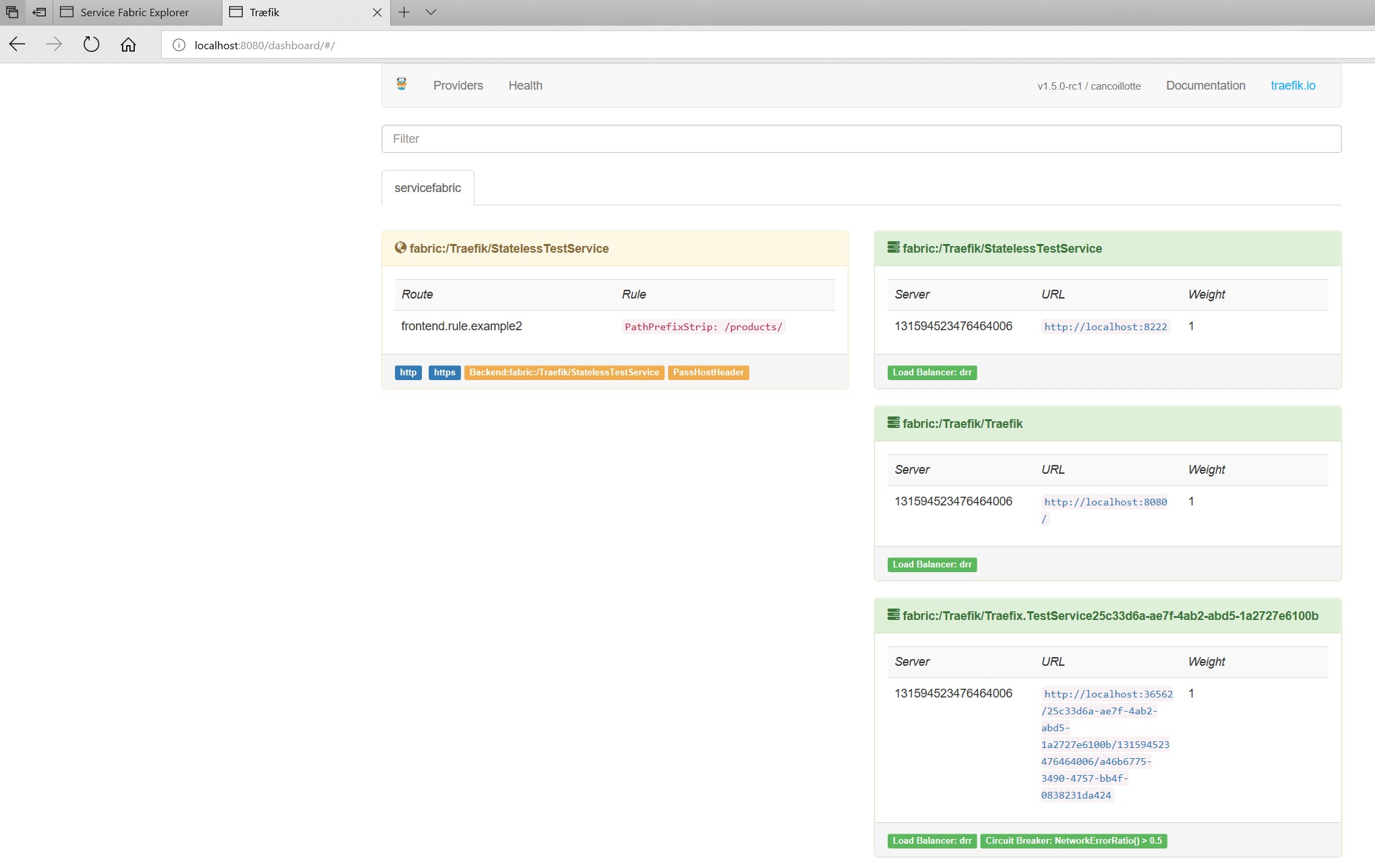
As you can see, the two microservices can be individually accessed through their endpoints but they can also be accessed through the traefik's endpoint localhost:8080. If I don't want to access the microservices directly, I can deny access to their ports on the Service Fabric Load Balancer.
Exposing a Service Fabric Application
Træfik uses the concept of labels to configure how services are exposed.
Labels allow you to define additional metadata for your services which Træfik can use to configure itself dynamically.
Adding Service labels
In order to assign labels for your service, you can add Extensions to your service's ServiceManifest.xml. Træfik will select any labels prefixed with traefik. Here is an example of using extensions to add Træfik labels:
Dynamically updating Service labels
Once you've deployed your service with some default labels, you may need to change the way Træfik is routing requests without redeploying. You can overwrite and add new labels to a named service using Service Fabric's Property Management API. Træfik will then pick up these new/updated labels and reconfigure itself.
Setting a label dynamically using curl
WARNING: The json provided in the body is case sensitive
Setting a label dynamically using sfctl
A future release of sfctl will allow you to operate with properties without make raw HTTP requests. There is an outstanding PR here if you wish to try this right away.
Available Labels
The current list of available labels is documented here.
Debugging
Both services will output logs to stdout and stderr. To enable these logs uncomment the ConsoleRedirection line in both ServiceManifest.xml files.
Once deployed, logs will be stored in the nodes Service Fabric folder. This will be similar to D:\SvcFab\_App\TraefikType_App1\log but may vary depending on your configuration.
Disclosure : These instructions are provided by Traefik development team and are provided as is. I've tried the same steps on my local box and it works like magic.
Over the next few days, I plan to use Traefik reverse proxy lot more in my production applications and will update here how it performs.
Cheers
Preetham Reddy, Cloud Solutions Architect at Tech FabricTech Fabric specializes in building web, mobile and cloud native application using Microsoft Stack (C#, .NET Core, Xamarin, Azure, SQL Service etc.,). If you need help with taking your on-premise application to cloud or convert your monolithic applications to microservices, we’d be glad to help you out. You can reach out to our sales team at contact@techfabric.com